-
Product Management
Software Testing
Technology Consulting
-
Multi-Vendor Marketplace
Online StoreCreate an online store with unique design and features at minimal cost using our MarketAge solutionCustom MarketplaceGet a unique, scalable, and cost-effective online marketplace with minimum time to marketTelemedicine SoftwareGet a cost-efficient, HIPAA-compliant telemedicine solution tailored to your facility's requirementsChat AppGet a customizable chat solution to connect users across multiple apps and platformsCustom Booking SystemImprove your business operations and expand to new markets with our appointment booking solutionVideo ConferencingAdjust our video conferencing solution for your business needsFor EnterpriseScale, automate, and improve business processes in your enterprise with our custom software solutionsFor StartupsTurn your startup ideas into viable, value-driven, and commercially successful software solutions -
-
- Case Studies
- Blog
How to Interview Your Ruby on Rails Developer
We at RubyGarage want to share our knowledge about how to interview a Ruby on Rails developer. Since our main purpose is to sell great code, we require great coders.
This article will come in handy for you when you need to test a Ruby on Rails programmer but aren’t sure what questions to ask the Rails interviewee. You can consider this article as a guide for how to interview a Ruby on Rails programmer.
We’re not going to include all the questions you could ask, as that would take more than one article. For example, we decided to omit questions about code idioms and cunning expressions in Ruby. Also, we don’t want to give away all the questions which we might ask during an interview with a developer. We don't want a developer to simply look for answers on the Internet, as our main purpose is to ensure that we check the developer's grasp of the language and framework. We merely want to see how a Ruby software engineer expounds their knowledge of the domain.
Now let's chalk out the structure of a Ruby on Rails interview. The article will be divided into several parts since we usually check separate domains of knowledge. Here is the structure we typically use:
- Ruby questions;
- Ruby on Rails questions;
- A pair programming task;
- A home task.
Now it’s time to start asking questions to your Ruby on Rails developer to find out what they know!
Ask Ruby Questions to Test a Web Developer
Why do we ask Ruby-related questions to a Ruby on Rails developer? Because the Rails framework is written in Ruby. This means that when we write code for Ruby on Rails, we’re using Ruby. The main issue we encounter with Ruby on Rails programmers is actually that they don’t completely understand the basics – the programming language itself. We want to hire forward-thinking software engineers who will create high-quality code, and so we want to assess their Ruby competence.
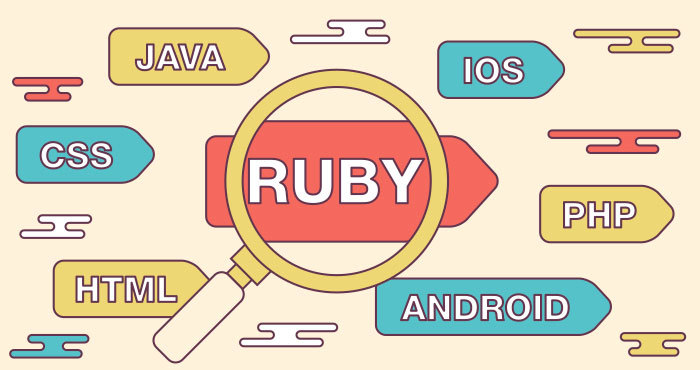
Our Ruby interview questions usually concern the Object Oriented Programming paradigm and object oriented design patterns. Class hierarchies, encapsulation, inheritance, and polymorphism are key concepts that every Ruby on Rails web developer should know well.
If a programmer correctly answers a list of questions similar to the ones below, then we move forward. Quality preparation for a job interview is important for a Ruby programmer. So if they can’t answer the following questions satisfactorily, then the interview is already over.
- What is a class?
- What is the difference between a class and a module?
- What is an object?
- How would you declare and use a constructor in Ruby?
- How would you create getter and setter methods in Ruby?
- Describe the difference between class and instance variables?
- What are the three levels of method access control for classes and what do they signify?
- What does ‘self’ mean?
- Explain how (almost) everything is an object in Ruby.
- Explain what singleton methods are. What is Eigenclass in Ruby?
- Describe Ruby method lookup path.
- Describe available Ruby callbacks. How can we use them in practice?
- What is the difference between Proc and lambda?
The Second Series of Ruby Questions: Business Applications
Knowing the basics isn't enough to work for RubyGarage or for any other serious web development company. A programmer should also be able to explain how to write code for business applications. Since Rack is a very popular interface that makes it possible to develop an application in Ruby, we ask specific questions about it. Here are four possible questions and challenges:
- What is Rack?
- Explain the Rack application interface.
- Write a simple Rack application.
- How does Rack middleware works?
The Third Series of Ruby Questions: Ruby Gems
Ruby is a very popular programming language and it has a huge community of developers who create numerous helpful libraries. We at RubyGarage love gems because they simplify and accelerate the work process. Third-party code helps us develop web applications quickly and smoothly.
In this part of the Ruby on Rails interview, we want to learn how a Ruby web developer perceives the basic structure of a gem library. A programmer will use multiple gems when building applications on the job, which is why it’s important for us to see if the developer can read and comprehend code written by other programmers. The interviewee should also describe RubyGems, which is a special system to create, implement, and share gems.
We have built up a series of four questions about Ruby gems:
- What is RubyGems? How does it work?
- How can you build your own Ruby gem?
- Explain the structure of a Ruby gem.
- Can you give me some examples of your favorite gems besides Ruby on Rails?
Ruby on Rails Interview Questions
Now it's time to plunge into the world of the Rails framework. A qualified developer should be familiar with the Model-View-Controller approach to building applications. The series of Ruby on Rails interview questions for experienced programmers is divided into three groups. First, we ask some general questions related to the Rails framework. Second, we want to see what the developer knows about routing, controllers, and views – the main parts of any business application.
And finally, the ActiveRecord-related questions let us test how the programmer understands the Model part of an application. In order to work efficiently, a developer should write as little configuration code as possible when making ActiveRecord models. We ask about the conventions used for implementing such logic as well.
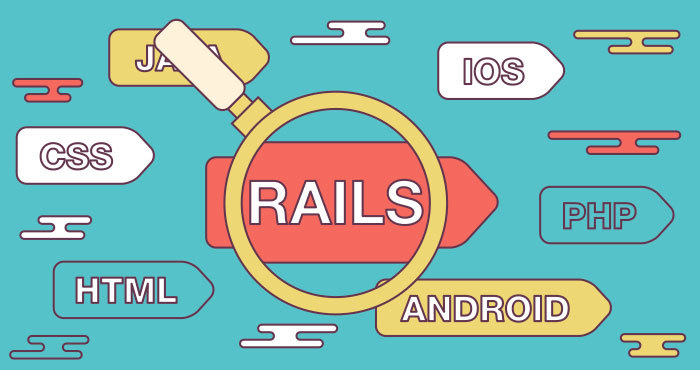
Top Ruby on Rails Interview Questions
- What is ActiveJob? When should we use it?
- What is Asset Pipeline?
- Explain the difference between Page, Action, Fragment, Low-Level, SQL caching types.
- What is a Rails engine?
Routing, Controllers, and Views
- Provide an example of RESTful routing and controller.
- Describe CRUD verbs and actions.
- How should you test routes?
- How should you use filters in controllers?
- What are Strong Parameters?
- What do we need to test in controllers?
- How should you use content_for and yield?
- How should you use nested layouts?
Active Record
- Explain the Active Record pattern.
- What is Object-Relational Mapping?
- Describe Active Record conventions.
- Explain the Migrations mechanism.
- Describe types of associations in Active Record.
- What is Scopes? How should you use it?
- Explain the difference between optimistic and pessimistic locking.
Ruby on Rails Questions: Security
We at RubyGarage know that a good application must be secure. We only hire programmers with a deep understanding of what types of attacks can be unleashed against a Ruby on Rails app. Besides possible attacks, a developer should also know everything about existing methods of protection. We want to see that a developer knows when to use such methods and what means of protection are good for what situations. As you will see in the suggested list of questions, we may be interested in not only Ruby on Rails security, but also in general web security concepts, such as the use of HTTPS instead of HTTP.
- Explain what is a sessions mechanism. How does it work?
- Describe cross-site request forgery, cross-site scripting, session hijacking, and session fixation attacks.
- What is the difference between SQL Injection and CSS Injection?
- How should you store secure data such as a password?
- Why do we need to use HTTPS instead of HTTP?
Questions About Automated Tests in Ruby on Rails
Writing code for production without automated tests is a bad approach to web development – especially when we code in Ruby, because the language itself expects web developers to write tests. Automated testing in Ruby on Rails helps reduce the workload for the Quality Assurance team, and this will greatly improve the workflow.
Our developers always create automated tests, which is why we demand our interviewees to understand automated testing. All Ruby web developers must have a clear view of why to use automated tests, when to write them, and what types of tests exist. On top of understanding testing methods, every Ruby on Rails developer should know how to create a testing case and should be familiar with testing guidelines and best practices.
Here are some questions we might ask a Ruby on Rails developer about testing:
- What is unit testing (in classical terms)?
- What is the primary technique for writing a test?
- What are your favorite tools for writing unit tests?
- What are your favorite tools for writing feature tests?
Ask the Ruby on Rails Programmer About Refactoring
We’ll also ask some Ruby on Rails questions related to code refactoring, because refactoring is an important step during the development of any application. Refactoring means that developers brush up the code they have already written to make it cleaner and more efficient. We want our Rails programmers to write high-quality code that is maintainable and expandable. When refactoring, RubyGarage programmers have to maintain a proper code structure and enhance the application’s performance without changing the behavior of the code.
If a developer knows how to reach high-level performance, then the team will be able to improve the application faster in the future according to the client's changing requests. The next series of Ruby on Rails interview questions will help you gauge a developer's ability to write elegant code. Don’t forget that these question can be altered to focus on your own primary development concerns:
- What is a code smell?
- What are your favorite tools to find code smells and potential bugs?
- Why should you avoid fat controllers?
- Why should you avoid fat models?
- Explain extract Value, Service, Form, View, Query, and Policy Objects techniques.
Give a Pair Programming Task
The next part of our Ruby on Rails interview always involves testing a programmer’s skills while paired with our senior web developer. We explain to the interviewee that this is necessary to unleash their mind. It doesn’t matter whether the interview is held online via Hangouts or Skype or whether it’s in-person, as we simply ask the interviewee to share their screen and start solving a task.
What is the purpose of a pair programming test? First of all, it gives us a look into the developer's line of thinking. Once a Ruby on Rails programmer gets the task, they should suggest some methods or means to resolve it. A pair programming task is very similar to a discussion, as our senior web developer helps the interviewee understand what solution might be not only workable, but best. Secondly, the pair programming tasks helps us determine a developer’s ability to work in a team. Below is an example of the tasks we might give during a Ruby on Rails interview.
Binary Gap
A binary gap within a positive integer N is any maximal sequence of consecutive zeros that is surrounded by ones at both ends in the binary representation of N. For example, the number 9 has binary representation 1001 and contains a binary gap of length 2. The number 529 has binary representation 1000010001 and contains two binary gaps: one of length 4 and one of length 3. The number 20 has binary representation 10100 and contains one binary gap of length 1. The number 15 has binary representation 1111 and has no binary gaps.
Write a function def binary_gap(n) that, given a positive integer N, returns the length of its longest binary gap. The function should return 0 if N doesn’t contain a binary gap.
For example, if N = 1041, the function should return 5, because N has binary representation 10000010001 and its longest binary gap is 5.
Assume that:
- N is an integer within the range [1..2,147,483,647].
Complexity:
- expected worst-case time complexity is O(log(N));
- expected worst-case space complexity is O(1).
Home Task for a Ruby on Rails Developer
This is the last task that we give to the interviewee. We want to figure out if a Rails developer is able to use multiple technologies for both frontend and backend web development. An interviewee should have a good grasp of many frontend tools, including the JavaScript programming language, its frameworks and libraries. Proficient knowledge of a markup language and stylesheets is definitely a must. We further include tasks to test a developer’s ability to write autotests using tools such as RSpec, Capybara, and similar.
The home task can vary. For example, we may ask a Rails developer to implement a task with React, Ember, or Backbone instead of Angular. The particular task we give may depend on a particular project on which the Rails developer will work if hired.
Once a developer resolves our home task and we check the result, we can know for sure if this programmer is ready to work with us or not. This programming task, to be completed solely by a job seeker, is very valuable to verify his or her skills.
Task Definition
I'm passionate about productivity. I want to manage my tasks and projects more effectively. I need a simple tool that helps me control my task flow.
Functional Requirements:
- I want to be able to sign in/sign up by email/password or Facebook;
- I want to be able to create/update/delete projects;
- I want to be able to add tasks to my project;
- I want to be able to update/delete tasks;
- I want to be able to prioritise tasks within a project;
- I want to be able to set a deadline for my task;
- I want to be able to mark a task as 'done';
- I want to be able to add comments to my tasks;
- I want to be able to delete comments;
- I want to be able to attach files to comments.
Technical Requirements
- It should be a WEB application:
- For the client side, use HTML5, CSS3, Bootstrap, JavaScript, AngularJS, and jQuery;
- For the server side, use Ruby on Rails.
- It should have client side and server side validation;
- It should work like a single-page WEB application and should use AJAX technology (load and submit data without reloading a page);
- It should have a user authentication solution. The user should only have access to his/her own projects and tasks (Devise, Cancancan);
- It should have automated tests for all functionality (models - RSpec, controllers - RSpec, acceptance/functional tests - RSpec + Capybara);
The result should be similar to the one in the screenshot below:
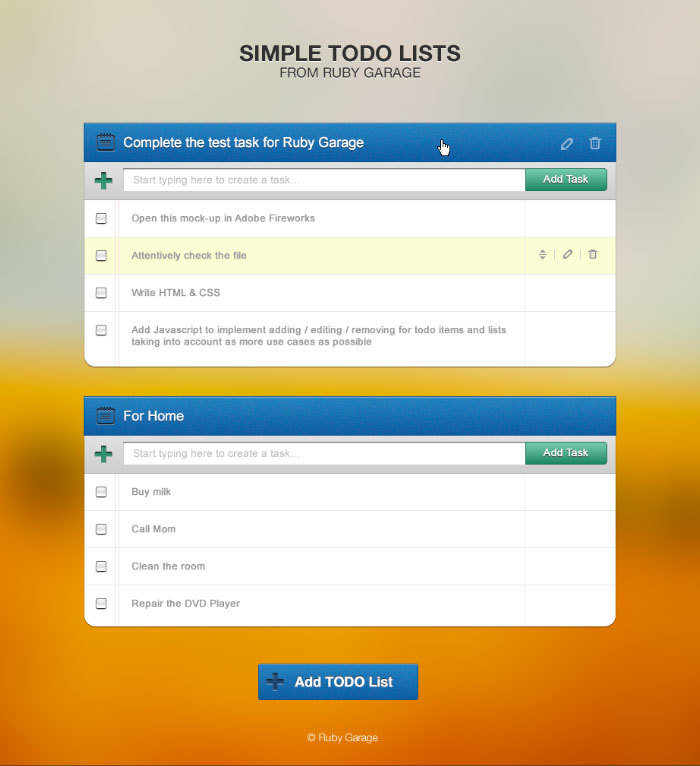
To Sum Up
There are many more Ruby on Rails interview questions that we might ask a programmer before we hire them. Our purpose was to show you how our interviews are organized at RubyGarage. The questions we ask are designed to analyze a programmer’s skills as fully as possible. Ask the right questions to your Ruby on Rails developers to make sure you hire a programmer who will be a great asset for your team!