-
Product Management
Software Testing
Technology Consulting
-
Multi-Vendor Marketplace
Online StoreCreate an online store with unique design and features at minimal cost using our MarketAge solutionCustom MarketplaceGet a unique, scalable, and cost-effective online marketplace with minimum time to marketTelemedicine SoftwareGet a cost-efficient, HIPAA-compliant telemedicine solution tailored to your facility's requirementsChat AppGet a customizable chat solution to connect users across multiple apps and platformsCustom Booking SystemImprove your business operations and expand to new markets with our appointment booking solutionVideo ConferencingAdjust our video conferencing solution for your business needsFor EnterpriseScale, automate, and improve business processes in your enterprise with our custom software solutionsFor StartupsTurn your startup ideas into viable, value-driven, and commercially successful software solutions -
-
- Case Studies
- Blog
Top 5 Design Patterns in Swift for iOS App Development
Released in 2014, Swift − Apple’s own programming language − is growing increasingly popular (it holds tenth place in RedMonk’s January 2018 rankings). Swift is a powerful tool that allows developers to create versatile applications for multiple operating systems (though it’s most frequently used to write applications for iOS).
Swift is a fairly new programming language, and many developers don’t know which design patterns they should use with it and how to implement them. Being able to use a relevant design pattern is a prerequisite to creating functional, high-quality, and secure applications.
We’ve decided to help by taking an in-depth look at the design patterns most widely used in Swift and showing different approaches to solving common problems in mobile development with them.
Design patterns: what they are and why you should know them
A software design pattern is a solution to a particular problem you might face when designing an app’s architecture. But unlike out-of-the-box services or open-source libraries, you can’t simply paste a design pattern into your application because it isn’t a piece of code. Rather, it’s a general concept for how to solve a problem. A design pattern is a template that tells you how to write code, but it’s up to you to fit your code to this template.
Design patterns bring several benefits:
- Tested solutions. You don’t need to waste time and reinvent the wheel trying to solve a particular software development problem, as design patterns already provide the best solution and tell you how to implement it.
- Code unification. Design patterns provide you with typical solutions that have been tested for drawbacks and bugs, helping you make fewer mistakes when designing your app architecture.
- Common vocabulary. Instead of providing in-depth explanations of how to solve this or that software development problem, you can simply say what design pattern you used and other developers will immediately understand what solutions you implemented.
Types of software design patterns
Before we describe the most common architecture patterns in Swift, you should first learn the three types of software design patterns and how they differ:
#1 Creational
Creational software design patterns deal with object creation mechanisms. They try to instantiate objects in a manner suitable for the particular situation. Here are several creational design patterns:
- Factory Method
- Abstract Factory
- Builder
- Singleton
- Prototype
#2 Structural
Structural design patterns aim to simplify the design by finding an easy way of realizing relationships between classes and objects. These are some structural architecture patterns:
- Adapter
- Bridge
- Facade
- Decorator
- Composite
- Flyweight
- Proxy
#3 Behavioral
Behavioral design patterns identify common communication patterns between entities and implement these patterns. Behavioral design patterns include:
- Chain of Responsibility
- Template Method
- Command
- Iterator
- Mediator
- Memento
- Observer
- Strategy
- State
- Visitor
Most of these design patterns, however, are rarely used, and you’re likely to forget how they work before you even need them. So we’ve handpicked the five design patterns most frequently used in Swift to develop applications for iOS and other operating systems.
Most frequently used design patterns in Swift
We’re going to provide only the essential information about each software design pattern – namely, how it works from the technical point of view and when it should be applied. We’ll also give an illustrative example in the Swift programming language.
#1 Builder
The Builder pattern is a creational design pattern that allows you to create complex objects from simple objects step by step. This design pattern helps you use the same code for creating different object views.
Imagine a complex object that requires incremental initialization of multiple fields and nested objects. Typically, the initialization code for such objects is hidden inside a mammoth constructor with dozens of parameters. Or even worse, it can be scattered all over the client code.
The Builder design pattern calls for separating the construction of an object from its own class. The construction of this object is instead assigned to special objects called builders and split into multiple steps. To create an object, you successively call builder methods. And you don’t need to go through all the steps – only those required for creating an object with a particular configuration.
You should apply the Builder design pattern
- when you want to avoid using a telescopic constructor (when a constructor has too many parameters, it gets difficult to read and manage);
- when your code needs to create different views of a specific object;
- when you need to compose complex objects.
Example
Suppose you’re developing an iOS application for a restaurant and you need to implement ordering functionality. You can introduce two structures, Dish and Order, and with the help of the OrderBuilder object, you can compose orders with different sets of dishes.
#2 Adapter
Adapter is a structural design pattern that allows objects with incompatible interfaces to work together. In other words, it transforms the interface of an object to adapt it to a different object.
An adapter wraps an object, therefore concealing it completely from another object. For example, you could wrap an object that handles meters with an adapter that converts data into feet.
You should use the Adapter design pattern
- when you want to use a third-party class but its interface doesn’t match the rest of your application’s code;
- when you need to use several existing subclasses but they lack particular functionality and, on top of that, you can’t extend the superclass.
Example
Suppose you want to implement a calendar and event management functionality in your iOS application. To do this, you should integrate the EventKit framework and adapt the Event model from the framework to the model in your application. An Adapter can wrap the model of the framework and make it compatible with the model in your application.
#3 Decorator
The Decorator pattern is a structural design pattern that allows you to dynamically attach new functionalities to an object by wrapping them in useful wrappers.
No wonder this design pattern is also called the Wrapper design pattern. This name describes more precisely the core idea behind this pattern: you place a target object inside another wrapper object that triggers the basic behavior of the target object and adds its own behavior to the result.
Both objects share the same interface, so it doesn’t matter for a user which of the objects they interact with − clean or wrapped. You can use several wrappers simultaneously and get the combined behavior of all these wrappers.
You should use the Decorator design pattern
- when you want to add responsibilities to objects dynamically and conceal those objects from the code that uses them;
- when it’s impossible to extend responsibilities of an object through inheritance.
Example
Imagine you need to implement data management in your iOS application. You could create two decorators: EncryptionDecorator for encrypting and decrypting data and EncodingDecorator for encoding and decoding.
#4 Facade
The Facade pattern is a structural design pattern that provides a simple interface to a library, framework, or complex system of classes.
Imagine that your code has to deal with multiple objects of a complex library or framework. You need to initialize all these objects, keep track of the right order of dependencies, and so on. As a result, the business logic of your classes gets intertwined with implementation details of other classes. Such code is difficult to read and maintain.
The Facade pattern provides a simple interface for working with complex subsystems containing lots of classes. The Facade pattern offers a simplified interface with limited functionality that you can extend by using a complex subsystem directly. This simplified interface provides only the features a client needs while concealing all others.
You should use the Facade design pattern
- when you want to provide a simple or unified interface to a complex subsystem;
- when you need to decompose a subsystem into separate layers.
Example
Lots of modern mobile applications support audio recording and playback, so let’s suppose you need to implement this functionality. You could use the Facade pattern to hide the implementation of services responsible for the file system (FileService), audio sessions (AudioSessionService), audio recording (RecorderService), and audio playback (PlayerService). The Facade provides a simplified interface for this rather complex system of classes.
#5 Template Method
The Template Method pattern is a behavioral design pattern that defines a skeleton for an algorithm and delegates responsibility for some steps to subclasses. This pattern allows subclasses to redefine certain steps of an algorithm without changing its overall structure.
This design pattern splits an algorithm into a sequence of steps, describes these steps in separate methods, and calls them consecutively with the help of a single template method.
You should use the Template Method design pattern
- when subclasses need to extend a basic algorithm without modifying its structure;
- when you have several classes responsible for quite similar actions (meaning that whenever you modify one class, you need to change the other classes).
Example
Suppose you’re working on an iOS app that must be able to take and save pictures. Therefore, your application needs to get permissions to use the iPhone (or iPad) camera and image gallery. To do this, you can use the PermissionService base class that has a specific algorithm. To get permission to use the camera and gallery, you can create two subclasses, CameraPermissionService and PhotoPermissionService, that redefine certain steps of the algorithm while keeping other steps the same.
Wrapping up
We’ve taken a close look at the five design patterns most frequently used in Swift. In this repository, you can find examples of how to implement other software architecture patterns in case you need them.
The ability to pick a design pattern in Swift that’s relevant for building a particular project allows you to build fully functional and secure applications that are easy to maintain and upgrade. You should certainly have design patterns in your skillset, as they not only simplify software development but also optimize the whole process and ensure high code quality.
If you want to stay updated on the most recent trends in mobile and web development, subscribe to our blog.

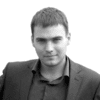