-
Product Management
Software Testing
Technology Consulting
-
Multi-Vendor Marketplace
Online StoreCreate an online store with unique design and features at minimal cost using our MarketAge solutionCustom MarketplaceGet a unique, scalable, and cost-effective online marketplace with minimum time to marketTelemedicine SoftwareGet a cost-efficient, HIPAA-compliant telemedicine solution tailored to your facility's requirementsChat AppGet a customizable chat solution to connect users across multiple apps and platformsCustom Booking SystemImprove your business operations and expand to new markets with our appointment booking solutionVideo ConferencingAdjust our video conferencing solution for your business needsFor EnterpriseScale, automate, and improve business processes in your enterprise with our custom software solutionsFor StartupsTurn your startup ideas into viable, value-driven, and commercially successful software solutions -
-
- Case Studies
- Blog
How to Separate Features for Different Organizations in a Rails App
When developing a multi-tenant Software as a Service application, designing the database layer is the first architectural problem you’ll have to address. But a database layer is only one part of the multi-tenant architecture.
When the same application instance is used by multiple organizations, otherwise called tenants, the app often provides identical core business functionalities to all of them. The main problem with the architecture layer that implements the business logic becomes evident when an organization wants more than just the core features.
As an emergency solution, your web development team can implement and deploy new features for all organizations. But will those other organizations ever need any of these new features? That’s questionable. Besides, if you decide to create subscription plans with extra features, then you’ll need to somehow restrict access to them.
So how can we approach development of a multi-tenant SaaS app when only a few organizations require additional functionality? We can’t just copy the app and extend it with new features individually for every new tenant, as doing so would break the multi-tenancy concept. Given that each application instance must be the same, we have to determine which features are available to which tenants.
We’ll review the two main techniques for separating feature stacks in a multi-tenant SaaS app. We’ll also name a library that your team can use to work on the business logic layer for a multi-tenant SaaS app. Although we’ll suggest solutions to separate feature stacks in a Rails-based SaaS application, the principles behind developing various feature stacks are similar no matter what backend framework you’re using.
Techniques for Working on Feature Stacks
Before we actually start developing a multi-tenant Rails-based SaaS app, we have to think about the following:
- How to alter functionalities so that changes for one organization can’t influence functionalities available to other organizations
- How to hide or show extra functionalities for organizations (this is especially important when implementing subscription plans)
When working on a multi-tenant SaaS app, we always have to address the first problem. As for the second problem, we may not need to focus on it. We only need to hide functionality if an application will provide additional features via subscription plans.
To develop multiple feature stacks, we can choose one of the following paths:
- Build a fully custom implementation. This approach gives us supreme flexibility, especially with regard to updates. A custom implementation can take one of two forms:
- A custom library that enables or disables features for organizations
- Feature packages that separate feature stacks into separate modules; in Ruby on Rails development, such modules are called engines
- Use an existing library. The Ruby ecosystem has plenty of libraries to restrict access to features for various user groups, but we’ll name the best and most popular libraries for feature separation.
The logic behind each of these three methods of dividing features is conditional statements. We must verify whether an organization has access to given functionality. Now let’s investigate each option for separating features in multi-tenant applications.
Custom Implementation of Feature Stacks
Implement Feature Toggles for Your App
A custom implementation of feature separation can be based on the feature toggles technique. Since each app is only one instance of a multi-tenant app, we can simply add or remove (toggle) features on the fly to show or hide certain features for different organizations. We can take advantage of the same feature toggles technique that’s often used in web development.
The feature toggles technique is chiefly used for A/B testing, for rolling out experimental features, and for testing new features on specific groups of customers. But feature toggles are also suitable for restricting access to features for organizations.
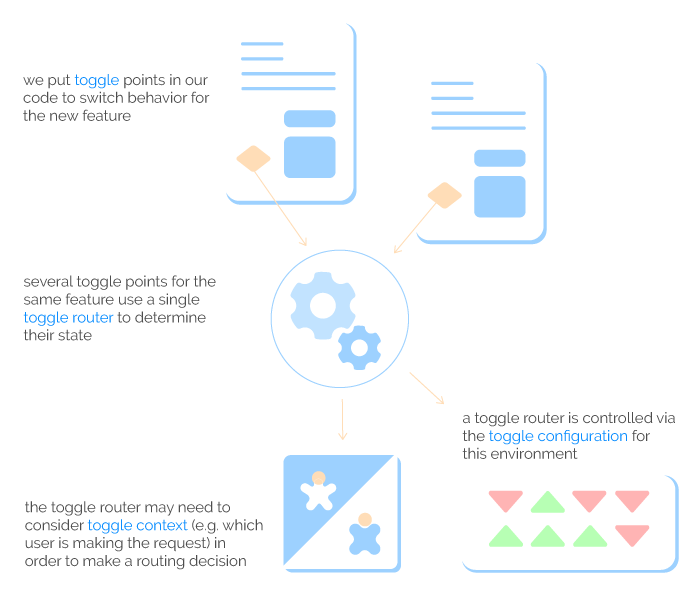
With feature toggles, we can dynamically adapt app functionality for each organization. With each request from a new organization, the app will change the view to show only features that are available to that organization.
To set up permissions for multiple organizations, we have to make changes in several parts of the application:
- Set up app configurations by enabling or disabling functionalities in controllers (in the case of Ruby on Rails).
- Reflect changes in the view part of the app (the graphical user interface).
Roughly speaking, the controller part of the application is responsible for the actual logic behind checks of an organization’s rights. The view part (templates) is an endpoint that runs the check and renders only those parts that are available to an organization.
In this article, we'll offer three tutorials on feature separation in a Rails application. In these tutorials, we'll use the Blog feature as an example and we’ll show you how to give access to this feature only to specific parties. Each tenant (organization) should be able to manage their own articles but shouldn’t have access to other tenants’ articles.
Let's start with the first tutorial, which shows a custom implementation of feature separation:
- Generate Blog with scaffold in the console:
- Generate Tenant, Feature, and Subscription models in the console:
- Modify the Tenant model app/models/tenant.rb
- Modify the Feature model app/models/feature.rb
- Modify the Subscription model app/models/subscription.rb
- Create a new file app/models/feature_toggle.rb with the class FeatureToggle
- Modify app/controllers/application_controller.rb
- Add tenants, features and subscriptions with the console:
- Modify app/controllers/articles_controller.rb
- Modify the link to show article in app/views/articles/index.html.erb
- Run the following code in the console:
- Create a new mountable engine in your project folder with the console command:
- Also, create an engines folder to store the engine:
- Modify Gemfile
- Modify the real values of engines/feature_blog/feature_blog.gemspec to avoid warnings.
- Run
- Next, add a small initializer to the engines/feature_blog/lib/feature_blog/engine.rb file:
- Generate an articles scaffold for your engine:
- Next, modify config/routes.rb:
- Replace the files in /engines/feature_blog/app/views/feature_blog/articles/ with files from ./app/views/articles/ and then modify engines/feature_article/app/controllers/feature_article/articles_controller.rb
Let's suppose that only HappinessCorp can show blog articles:
Finally, open a browser and go to http://happinesscorp.lvh.me:3000/articles. Once you're logged into a happinesscorp account, you can see Blog links. If you change happinesscorp to feelgoodinc in the URL – http://feelgoodinc.lvh.me:3000/articles – the link becomes hidden. Even if the tenant inserts the link to an existing article, they’ll be redirected to http://feelgoodinc.lvh.me:3000/articles and see the message “You don’t have an access to this feature.”
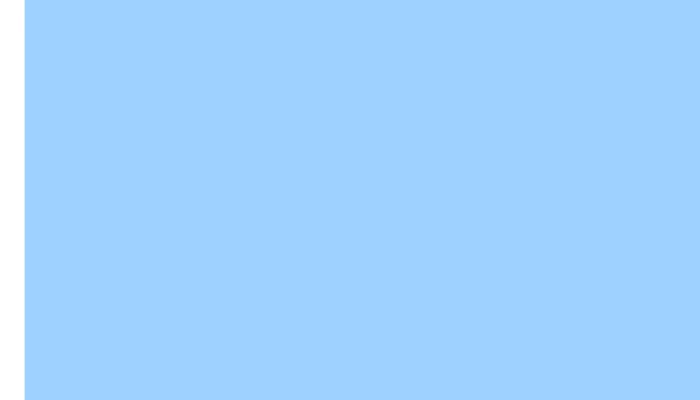
As we can see, in order to reflect changes in the view we can simply use if statements: if a tenant is allowed to use a certain feature, then the additional layout is rendered on the screen.
Using checks directly in templates works great when a complete feature must be made available for a given organization. But when the same feature is implemented differently for different organizations, then it’s better to move the logic to a lower-level implementation.
When working on a Rails-based web application, we can basically create our own library that will help us determine an organization’s permissions.
Next, let’s review another custom way to organize features using Rails engines.
Encapsulate Feature Stacks in Engines
Another way to separate features is by wrapping them in engines – small Ruby on Rails applications. The basic way this works is the same as in the previous example. When a user of a certain organization signs in and is authorized to use a feature, the application requests the proper engine.
Here's a step-by-step tutorial on how to separate features by wrapping them into engines:
At this point, nobody has access to your articles functionality. Grant access to the article feature to happinesscorp. To do that, run the following command in the console:
Run the application. Now only happinesscorp has access to the article feature at this URL: http://happinesscorp.lvh.me:3000/articles.
Using the Rails engines is beneficial as we can create typical, completely independent feature stacks. We recommend using engines as it’s a tad easier to configure engines than to use the previous approach.
Using Rails engines to encapsulate features, however, also has downsides. One disadvantage is that you may end up having too many engines. Managing dozens of engines that implement different functionalities can create additional management overhead.
But this approach is beneficial when you develop a white label application. A white label application looks like it’s fully customized for separate tenants, but it’s still essentially the same for all of them. Since a given organization might want to have a rather advanced feature stack, you might consider encapsulating that stack in an engine.
So far, we’ve discussed two approaches for a custom implementation of the feature toggles technique: with and without Rails engines. But with Ruby on Rails-based applications, we can also benefit from using ready libraries when developing feature toggles. Using existing libraries makes it simpler to work on different features. We’ll discuss two libraries for Rails-based multitenant apps in the following section.
Use a Ready Library to Separate Features for Organizations
If you don’t want to spend time writing custom logic, you might opt for an existing library. The Ruby and Rails ecosystem counts more than a dozen libraries that are suitable for developing feature toggles.
- Flipper is a beginner-friendly gem that makes it easy to switch features on and off. Flipper has many adapters including for Mongo, Redis, Sequel, Cassanity, and ActiveRecord.
- Rollout is a relatively old yet still supported Ruby library that was designed to deliver functionalities to user groups such as admins, registered users, and new users. With Rollout, we can also manage features for organizations.
- LaunchDarkly SDK for Ruby is an SDK for an advanced feature flag tool that allows you to easily create feature flags, create custom user roles and custom policies for those roles, gradually roll out features, perform A/B testing, and more. LaunchDarkly provides SDKs not only for Ruby but also for other programming languages including PHP, Python, and Go.
- Feature Flipper is a small library that helps you restrict certain pieces of code to certain environments.
- Setler implements the Feature Flag pattern and allows you to add settings to individual models.
- Bandiera is a feature flagging solution that can be used with many programming languages and frameworks including Ruby and PHP since its communication is implemented with the help of a simple REST API.
We’ll use Flipper to develop feature toggles in our example.
Flipper was inspired by the Rollout gem, but Flipper proves a more flexible solution that Rollout. With Flipper, we’re not restricted to using Redis to store features. In addition to Redis, it’s possible to use other types of storage including but not limited to:
- Relational databases with connectors
- ActiveRecord, object-relational mapping designed for Ruby on Rails
- Sequel, a toolkit that provides access to relational databases
- MongoDB, a document-oriented database
- Cassandra, a NoSQL database
Flipper introduced several concepts, namely the actor, feature, and gate. With a multi-tenant app, an actor is an organization that uses the app, and gates are responsible for checking if a feature can be used by an organization. The Flipper library also implements the adapter pattern, and you can easily extend the library with your own adapter to work with a specific relational database just like one of our RubyGarage developers did with Sequel.
Here’s our final tutorial that explains how to separate features with the Flipper gem.
- Modify the Gemfile:
- Then run
- Modify config/initializers/flipper.rb
- Modify config/routes.rb
- Modify app/models/tenant.rb
- Update engines/feature_blog/app/feature_blog/controllers/articles_controller.rb
- Then update engines/feature_blogapp/views/feature_blog/articles/index.html.erb
- Next, add helper_method :current_tenant in the ApplicationController.
- Update config/routes.rb
- Finally, run the server with
Register the Blog feature for the actor happinesscorp via the Flipper UI.
Aslo, register the feature_blog feature for the actor happinesscorp.
As a result, you’ll get the same behavior as in the previous example, only with a custom solution.
We’ve provided you with enough information to get started with Flipper. If you want to see a more detailed explanation of how Flipper works, you can check out a review written by the creator of the library.
So which approach – a custom approach or a ready library – should you choose for separating features in your own multi-tenant Rails-based application? There’s no definite answer, as the same approach won’t suit all Software as a Service applications. To get the most flexibility for your particular application, admittedly, it’s best to write fully custom logic to check each organization’s rights and show the appropriate features. But if time is expensive or in short supply, using Flipper is also a viable solution.
If you’re interested in getting even more useful articles on this topic, subscribe to our newsletter to receive a monthly update on our posts!