-
Product Management
Software Testing
Technology Consulting
-
Multi-Vendor Marketplace
Online StoreCreate an online store with unique design and features at minimal cost using our MarketAge solutionCustom MarketplaceGet a unique, scalable, and cost-effective online marketplace with minimum time to marketTelemedicine SoftwareGet a cost-efficient, HIPAA-compliant telemedicine solution tailored to your facility's requirementsChat AppGet a customizable chat solution to connect users across multiple apps and platformsCustom Booking SystemImprove your business operations and expand to new markets with our appointment booking solutionVideo ConferencingAdjust our video conferencing solution for your business needsFor EnterpriseScale, automate, and improve business processes in your enterprise with our custom software solutionsFor StartupsTurn your startup ideas into viable, value-driven, and commercially successful software solutions -
-
- Case Studies
- Blog
How to build a Progressive Web App with React & Apollo Client
This guide is for developers who want to make a web application that looks and behaves like a native app.
In this article, we’ll show how to build a progressive web app (PWA) using React and Apollo client. Our PWA will launch from the home screen, run in a separate window, send push notifications, and even work offline.
What’s a PWA?
A progressive web app is a modified website that looks like and gives the feel of a native app. These apps are called progressive due to their broad functionality and features such as fast response times, offline capabilities, and cross-platform compatibility. This makes development and maintenance much easier, as you don’t need to register a PWA in app stores (though you can if needed). Not surprisingly, big and famous corporations like Starbucks, Twitter, Uber, Pinterest, Spotify, and others choose PWAs over native apps.
The key characteristics of a PWA make up the acronym FIRE:
- Fast. Data exchange is fast, and a PWA’s UI is smooth and responsive.
- Integrated. PWAs feel natural to users and look like any other app.
- Reliable. A PWA launches fast and always displays content, even when the network connection fails.
- Engaging. The user experience is immersive and comfortable.
PWAs are gaining popularity due to a long list of benefits. Let’s dive deeper into the matter to learn about PWA’s advantages and disadvantages.
Advantages of progressive web apps
They’re fast
As a PWA caches resources, PWA pages load at a glance, affording a considerable advantage for any company. Users will definitely come back for such an experience. Moreover, using an app shell, web app manifest, and service worker, PWAs tend to have the speed of native apps.
They’re affordable
Any user with any device can access a PWA via a URL. PWAs download onto a device quickly, and they don’t need as much storage space as apps do.
PWAs are also cheaper and faster to build than native apps. All that a developer must know is HTML, CSS, and JavaScript.
They can work offline
Your users will never get a no connection error, as PWAs can use a service worker API that keeps pages cached for offline use. So if users don’t have an internet connection, they’ll still see your app’s content.
Disadvantages of progressive web apps
Despite a long list of benefits PWAs provide, there are also some drawbacks you need to be aware of.
They lack app features
Due to their limited functionality, PWAs have restricted access to device hardware and user data (calendars, contacts, alarms, social media profiles).
They’re difficult to track
As PWAs work offline, it’s hard to monitor and evaluate their performance and metrics. This may lead to halts in PWA maintenance and operations.
They conflict with iOS
Unfortunately, push notifications fall into the category of PWA features that are not available to iOS users. In addition, iOS limits the amount of storage space available to PWAs, so cached files for offline use are available for only two weeks.
Challenges
In our case, we faced three challenges: we wanted our PWA to look like a native app, be able to work offline, and be able to send push notifications. To solve these challenges, we decided to create a progressive web app with React and Apollo Client. Let’s take a look at the advantages of this choice.
Why Apollo Client?
Apollo Client is the best option when integrating React apps with GraphQL APIs. When you finish writing a GraphQL query, Apollo Client will start requesting and caching the data as well as updating the UI of the PWA. With Apollo Client, you can add sophisticated features hassle-free.
When building a progressive web app with React, Apollo Client lets you:
- Fetch declarative data
- Enjoy useful tools for developers: TypeScript, Chrome DevTools, VS Code
- Benefit from brand-new React features such as hooks
- Add Apollo to a JavaScript app
- Share your knowledge and life hacks with other developers in the open-source community
#1 How to make a PWA look like a native app
In order to get rid of the browser-looking UI, we’ll use the web app manifest. This is a JavaScript Object Notation (JSON) file, an open and lightweight data interchange format for storing and transporting data. It will tell the browser how to behave when the PWA is installed on the user’s device.
Typically, a basic web app manifest includes:
name – The name of the app that users will see in the request to add the PWA to the home screen
short_name – The short name of the app that users will see on the home screen
background_color – The color of the splash screen when the user launches the PWA
display - Customizes the browser UI (We opted for standalone, which means that our PWA opens in a new window, changing the standard browser UI and hiding the address bar.)
scope – Gathers the set of URLs and helps you understand when the user has left the app
start_url – The URL that loads when the PWA launches; directs the user to the starting page, not the page where they added the PWA to the home screen
theme_color – Defines the color of the toolbar
icons – The set of icons that are used on the home screen, app launcher, task switcher, and splash screen
The result
As a result, we get the following web app manifest for our PWA:
Let's look at how the application looks in a browser:
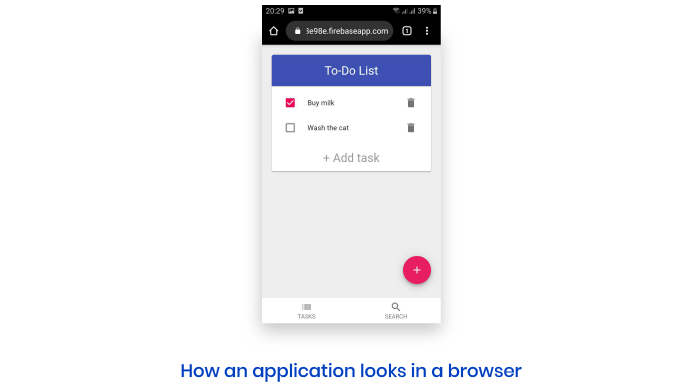
Next, we get an app that is functioning on a phone and looks like a native app with the icon:
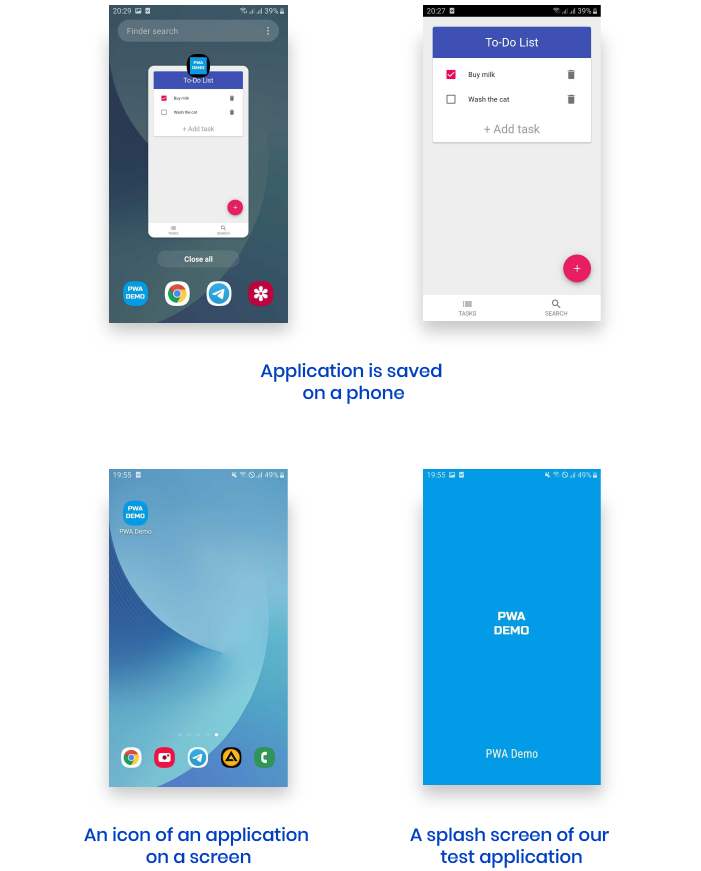
#2 Offline first
Offline first, or cache first, is a famous strategy for delivering content to users. This is how it works: if a resource is cached or available offline, we’ll try to get it from the cache. If the resource is not cached, we’ll download it from the server and add it to the cache.
Cache
To open a PWA while offline, we need to have its files cached: JavaScript, HTML, CSS, images, etc. This is when a service worker can help. We’ve applied a service worker to add files to the cache and show them to users when needed.
What we used
We used create-react-app with a built-in service worker. To make everything work, we changed serviceWorker.unregister() to serviceWorker.register() in index.js. After this, all files will be cached, so we can use the PWA without an internet connection and browse static pages.
Offline queries
In order for users to see previously loaded content while they’re offline, we also need the cache. But this time we’ll deal with server responses, not PWA files. The thing is, Apollo Client already stores responses in its cache. However, if you reload the page, everything will disappear. To work around this, we used apollo-cache-persist to save the cache in local storage. Now, when the PWA launches, the cache from local storage initializes the Apollo cache. In this way, users will see the app’s content when they don’t have an internet connection.
We decided to add a loader that users will see while the local storage cache initializes the Apollo cache.
Offline mutations
It looks like everything already works fine, but what if a user wants to post something or add a task to a to-do list while offline? Using Apollo, we can trick users and show that their post or task has been added offline. However, the real request will be sent when the connection is re-established.
We used an optimistic response in Apollo Client to make it look like a post or task has been added. The user is shown a fake optimistic response before the real response comes from the server. When the internet connection is re-established, Apollo will update the cache with the actual data.
Here’s how it works:
The code above shows the optimistic response to the user, but we still need to send the request. To fulfill this goal, we used a set of libraries:
- apollo-link-retry to send the request one more time in case something goes wrong
- apollo-link-queue to queue all offline requests
- apollo-link-serialize to use along with the apollo-link-queue when two mutations — for instance, adding a task and deleting it in offline mode — will be executed simultaneously, which can lead to errors
This is what we get as a result:
We want to record the offline mutation data in local storage and use, create, and execute new mutations based on the initial mutations when the user is online again.
Here’s the custom link for that:
We need to add the link above to this chain:
After that, we get the mutation data while initializing the component. We add it straight in the root component:
#3 Push notifications
The last thing we’re going to do is implement push notifications. We decided to do that via Firebase. We created a separate service worker firebase-messaging-sw.js and placed it in public.
Here is the result:
After that, you need to initialize Firebase in index.js.
Finally, we requested permission to send notifications to users when it’s most relevant. We aren’t going to send them at the start, as it’s irritating and users simply decline the request. The best practice is to send a request after the user has performed some action in the PWA and gotten positive feedback. At this point, the user is pleased and is ready to accept the request to send notifications. Alternatively, you can add a possibility to turn notifications on in the application's settings.
Summing up
A progressive web app built with React and Apollo client is a good alternative to a native app, especially when the native features of the web platform and browser API can cover your functionality. The outstanding advantage is that a PWA is still a web app, so we still can transform it into a mobile app hassle-free without rewriting the client part. Additionally, you can add your PWA to the App Store and Google Play Store if needed.

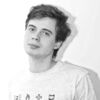
