-
Product Management
Software Testing
Technology Consulting
-
Multi-Vendor Marketplace
Online StoreCreate an online store with unique design and features at minimal cost using our MarketAge solutionCustom MarketplaceGet a unique, scalable, and cost-effective online marketplace with minimum time to marketTelemedicine SoftwareGet a cost-efficient, HIPAA-compliant telemedicine solution tailored to your facility's requirementsChat AppGet a customizable chat solution to connect users across multiple apps and platformsCustom Booking SystemImprove your business operations and expand to new markets with our appointment booking solutionVideo ConferencingAdjust our video conferencing solution for your business needsFor EnterpriseScale, automate, and improve business processes in your enterprise with our custom software solutionsFor StartupsTurn your startup ideas into viable, value-driven, and commercially successful software solutions -
-
- Case Studies
- Blog
Clean Architecture of Android Apps with Practical Examples
Writing quality code for medium and high-complexity applications requires considerable effort and experience. An application should not only meet the customer’s requirements but also be flexible, testable, and maintainable.
As a solution to these challenges, Robert C. Martin, also known as Uncle Bob, came up with the Clean Architecture concept in 2012.
In this article, we’ll review the concept of Clean Architecture in Android applications and show several code examples.
What is Clean Architecture?
Clean Architecture combines a group of practices that produce systems with the following characteristics:
- Testable
- UI-independent (the UI can easily be changed without changing the system)
- Independent of databases, frameworks, external agencies, and libraries
The dependency rule is the overriding rule that makes Clean Architecture work. It says that nothing in an inner circle should depend on anything in an outer circle. In particular, application and business rules shouldn’t depend on the UI, database, presenters, and so on. These rules allow us to build systems that are simpler to maintain, as changes in outer circles won’t impact inner ones.
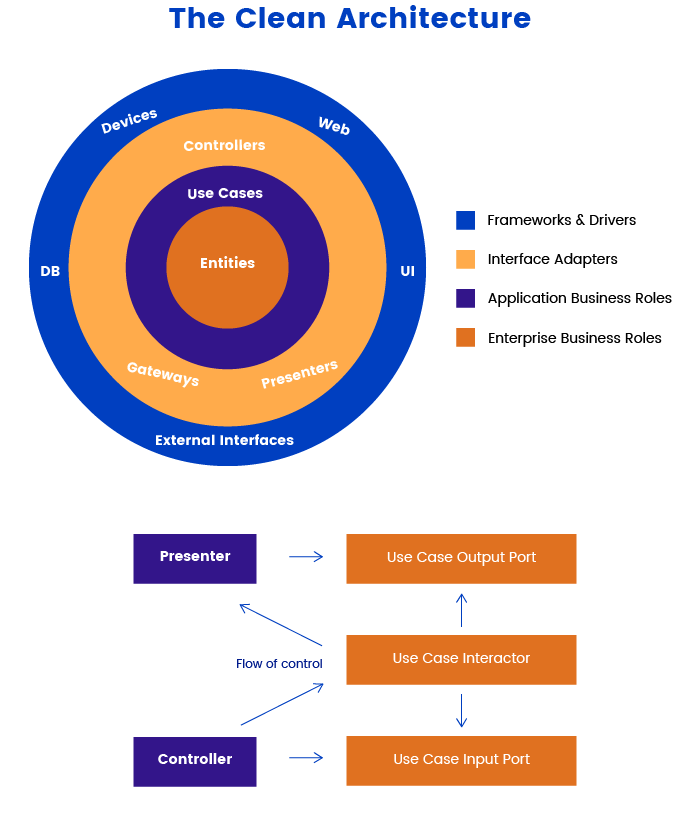
The original Clean Architecture scheme is rather confusing and often leads to misunderstanding of the main principles of the approach.
The following scheme is much easier to understand, as it’s presented from the UI to the backend or database:
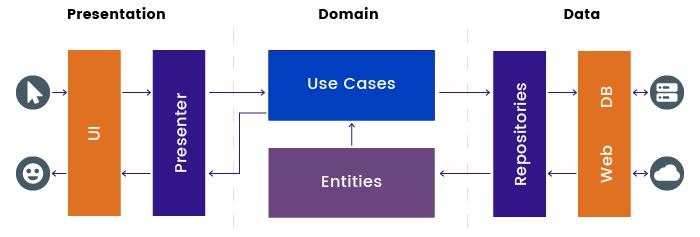
Here’s a brief definition of some terms from the images above to help you get more familiar with this approach:
- Entities are enterprise-wide business rules that encapsulate the most general business rules and also contain Data Transfer Objects (DTOs). When something external changes, these rules are the least likely to change.
- Use cases are also called interactors and stand for application-specific business rules of the software. This layer is isolated from changes to the database, common frameworks, and the UI.
- Interface adapters convert data from a convenient format for entities and use cases to a format applicable to databases and the web, for example. This layer includes Presenters from MVP, ViewModel from MVVM, and Gateways (also known as Repositories).
- Frameworks and drivers are the outermost layer, which consists of the web framework, database, UI, HTTP client, and so on.
Now we can look at the pictures above with deeper understanding. In the first stage, the user opens the screen and the system requests data from the Presenter (or ViewModel). In the Presenter layer, the UseCase makes a request to the Repository whether there’s project data in the database.
If the result is positive, the Repository will create the project’s DTO and will return the data to UseCases. If there is no project data in the database, then the Repository will make a request in the net and save the data to the database. A UseCase, in turn, returns data to the Presenter, which returns this data to the UI where the information is rendered.
As you can see in the image, an Android application with Clean Architecture gathers the layers into three modules:
- Presentation, which includes the UI, Presenter, and ViewModels
- Domain, which includes Entities and Interactors
- Data, which includes Databases and Rest clients
Technologies for implementing Clean Architecture in Android apps
Here’s a short list of technologies used in this example:
- Kotlin ‒ A statically typed programming language for modern multi-platform applications; Google rates Kotlin a first-class language for writing Android apps
- Dagger 2 ‒ A fully static, compile-time dependency injection framework for both Java and Android
- RxJava 2 ‒ Reactive Extensions for the Java Virtual Machine (JVM) – a library for composing asynchronous and event-based programs using observable sequences for the JVM
- Retrofit 2 ‒ A type-safe HTTP client for Android and Java
Along with the Clean Architecture approach, the following Android architecture components will also be used in the application:
- Room ‒ Provides an abstraction layer over SQLite to allow for more robust database access while harnessing the full power of SQLite
- ViewModel ‒ Designed to store and manage UI-related data in a life cycle-conscious way
- LiveData ‒ An observable data holder class that, unlike a regular observable, is life cycle-aware, meaning it respects the lifecycle of other app components such as activities, fragments, and services
- Paging Library ‒ Makes it easier for you to load data gradually and gracefully within your app’s RecyclerView
Clean Android architecture approach in practice
Now let’s see how to implement Clean Architecture in Android applications.
To start, we need to create three modules: Presentation (an app in our case), Data, and Domain.
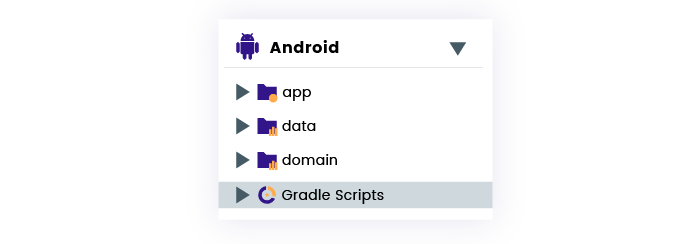
Domain
In this module we’ll place all objects that will interact with other layers.
Data Transfer Objects (DTOs) are usual Kotlin data classes:
The Repository is an interface that describes incoming parameters and the type of the object returned. Below, you can see an example of ProjectRepository:
ViewModel interacts with UseCases. It forms an object with the necessary parameters described in UseCase and makes a request which returns either an expected result or an error. UseCase acts not only as an intermediary between the ViewModel and Repository but can also request data from various Repositories and combine it or apply additional calculations. Developers can create several types of UseCases based on the base class for different purposes. For example, GetProjectListUseCase:
Data
The Data module includes RestClient, Database, adapters, and so on.
Repository implementation
Implementation of a repository provides access to data with the ability to select a data source depending on the conditions.
Adapters
The main task of adapters is to convert the entities used by the database and network clients to Domain module entities and back. This conversion has both pros and cons:
- Any data changes in one layer don’t affect other layers
- Third-party dependencies as well as annotations required for a library don’t fall into other layers
- There’s a possibility of multiple duplications
- While changing the data you have to change the mapper
A detailed RestClient and AppDatabase implementation is provided in the project repository.
Presentation
The Presentation module includes ViewModels and the UI. In addition, we connect all dependencies from our DI in this module.
In order to avoid memory leaks when using UseCases, we need to unsubscribe from all UseCases. That’s why we use ViewModel for simplified life cycle processing. For even more convenience, we’ll create an abstract BaseViewModel class. After that, we’re able to create a subclass where all UseCases will be driven to the base constructor. Finally, we’ll unsubscribe from all UseCases in ViewModel.
In order to connect the dependencies in ViewModel, the developer needs to create ViewModelFactory, which will get all required dependencies in the constructor with the help of @Inject annotation. Then this model will create the required ViewModel with these dependencies in the create function.
With the help of the ready-made ViewModelFactory pattern made in Activity with DI, we create the ViewModel. After that, we can request and observe data coming into the ViewModel.
PagingLibrary with Clean Architecture
If you want to use PagingLibrary with Clean Architecture in an Android application, you’ll face a problem because they both use their own data sources. As it’s impossible to make a request to the network or database in the Presentation layer, you’ll need to use data sources described in the Data layer through the ViewModel. In this case, you just need to delegate all requests from PagingLibrary to the ViewModel.
As in our case there’s no need for PagingLibrary to request data in another thread (UseCases control this themselves), we can create a MainThreadExecutor that will be used for the setBackgroundThreadExecutor function and the setMainThreadExecutor function.
When using the UseCases described above, take into account that the execute method can be called several times. For instance, when entering a search phrase on the screen, a request will be sent to the network after each entered character and short debounce. And as requests are made asynchronously, the result of the first request may come last. This can lead to inconsistency between the text entered and the data found. This problem might be solved by increasing the debounce time, but sometimes that can even hurt the user experience more. The best solution in this case is to make unsubscribing from the previous request compulsory when making a new request.
When we use Single Repository and RxJava patterns, a problem with error processing appears. When an error occurs in one of the requests, the data may not come in time from the database and UseCase will return an error. To solve this issue, you can use the RxJava mergeDelayError method instead of the usual merge. In this case, when we observe some changes in the database we won’t get an error at all as onComplete won’t be called.
The problem will be solved by separating one UseCase into two. The first one will command the repository to make a request and save the received data to the database; the second will call back the required data from the first request and will observe all changes in the database. This way we can always call data back from the database and process the network error.
Key takeaways
To sum up, Clean Architecture is a great solution for building medium and high-complexity applications that will receive further support. It’s compatible with MVP and MVVM and works well with Android Architecture Components. Using this architecture for building simple apps may lead to overengineering, however.